React Best Practices: HOCs, Async Patterns and Testing Strategies
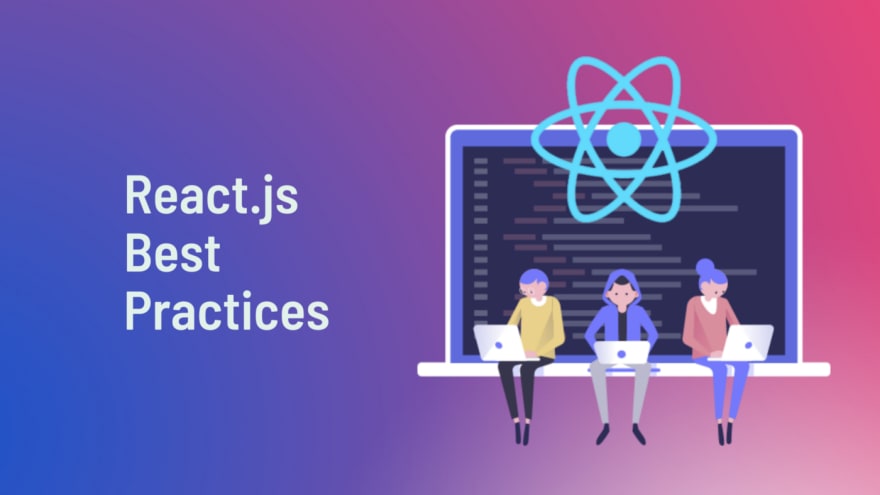
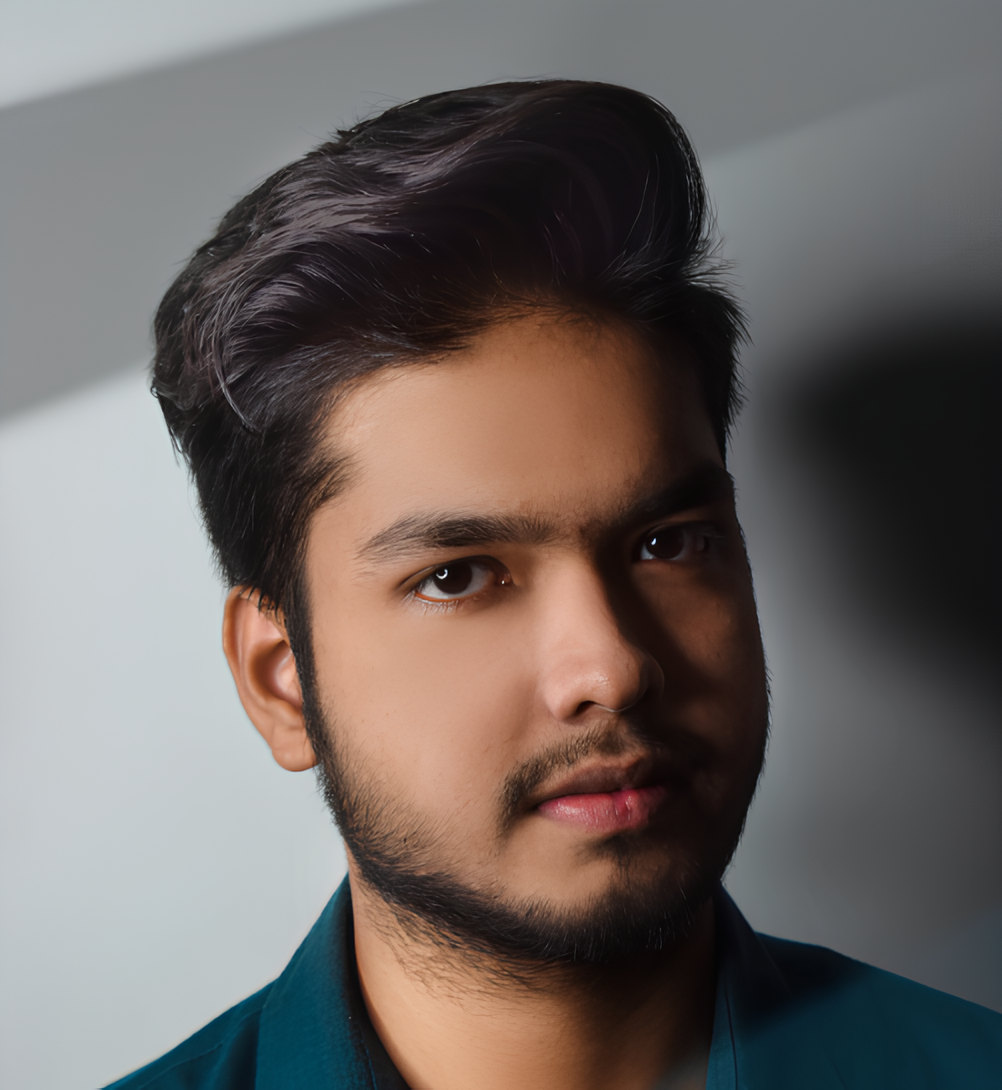
Hanzala
25 min read ⋅ Oct 10, 2023
Mastering React Design Patterns for Robust Applications
When venturing into the world of modern web development, the importance of design patterns becomes ever clearer. For those immersed in React — the eminent JavaScript library for user interface development — leveraging these patterns is paramount. This guide dives deep into React design patterns, shining light on their significance in sculpting efficient applications.
Why React Design Patterns?
- Structured Codebase: Dive into React, and you’ll see an ocean of components and modules. React design patterns act as a beacon, guiding developers in architecting their code efficiently.
- Scalable Solutions: For ever-evolving React projects, scalable solutions are indispensable. These patterns give a roadmap to adapt to this growth seamlessly.
- Component Modularity: Reusable components are the bedrock of React. With patterns, these components are molded to be versatile, amplifying efficiency.
- Optimized Performance: Every millisecond counts in web application performance. React patterns come equipped with methodologies to boost rendering speed and application responsiveness.
- Tackling React Challenges: Every React developer encounters hurdles like state management or data fetching. Patterns offer battle-tested strategies, saving both time and brainpower.
Dive into Component Composition and the Container-Presentation Pattern
Component Composition is the unsung hero behind powerful React applications. By emphasizing smaller, precision-focused components, allows the creation of complex UI elements.
Understanding Component Composition:
Imagine a digital puzzle. Each piece, representing a component, plays a unique role. For a social media app, instead of one colossal component managing everything, we have miniature ones — “UserAvatar,” “PostContent,” and “CommentsSection.” This promotes reusability and pinpoints specific roles for each.
Unveiling the Container-Presentation Pattern:
Often termed as “smart-dumb” or “containers-presentational” components, this pattern takes component composition a notch higher.
- Container Components: These are the brainy ones. Tasked with business logic, data management, and interactions, they rarely deal with UI elements.
- Presentation Components: These are the artists, purely focusing on the visual finesse and behaviour of UI components.
Advantages:
- Clearer Responsibilities: With a stark distinction between logic and UI, it’s easier to navigate, modify, and test individual components.
- Enhanced Reusability: UI elements can be reused seamlessly, sans any data or logic binding.
- Simplified Testing: Test data management without entangling with complex UI rendering.
- Concurrent Development: A harmonious workspace where designers and developers can coexist, working on UI and logic simultaneously.
Implementation Guide:
- Determine the logic and state requirements.
- Chisel a container component to manage data and states.
- Craft presentation components that rely on data from the container through props.
- Ensure smooth data flow and event handling between the two.
Practical Implementation:
Take, for instance, a user profile page. The “UserProfileContainer” manages data, while the “UserProfile” takes care of rendering.
// UserProfileContainer.js
...
class UserProfileContainer extends Component {
...
render() {
return <UserProfile user={this.state.user} isLoading={this.state.isLoading} />;
}
}
...
// UserProfile.js
...
const UserProfile = ({ user, isLoading }) => {
...
};
...
By weaving together Component Composition and the Container-Presentation pattern, React applications are transformed. Not only does it simplify management, but it also ensures applications are primed for future growth.
Efficient State Management in React using Redux
Navigating the intricate maze of state management in React can be a daunting endeavor, especially as applications expand and evolve. Redux emerges as a shining beacon, offering a streamlined method to handle state, ensuring code remains organized and free of tangles. Let’s journey through Redux’s pivotal role in refining state management within React applications.
Why is State Management Crucial in React?
Every React component is like an individual, each with its own set of states governing behaviour and presentation. But as these components interact and intermingle, sharing data across the expansive application landscape, the standalone state management within each becomes problematic. Issues like ‘prop drilling’ arise, complicating the code and making debugging an uphill battle.
Discovering Redux: The State Management Maestro
Redux, a stalwart in the JavaScript universe, is the definitive state container. When married to React, it offers centralized state management, ensuring every component accesses consistent data from a singular store. The brilliance of Redux lies in its foundational principles:
- Unified Data Hub: Often called the “store”, Redux harbors the entirety of the application state within a single JavaScript object, mitigating discrepancies.
- Immutable State: The Redux state is set in stone. Instead of direct modifications, components communicate their intentions via ‘actions’. Then, ‘reducers’ take the stage, crafting a fresh state.
- Predictable Alterations with Pure Functions: The reducers, in their purest form, accept the current state and dispatched action, churning out a new state, guaranteeing consistency and easing testing.
Unraveling Redux’s Key Concepts:
- Actions: These are the messengers, encapsulated within JavaScript objects, depicting potential state alterations. They proudly bear a ‘type’ property, signifying the change in nature.
- Reducers: The craftsmen of Redux, these pure functions carve out the new state by analyzing the current one and the received action.
- Store: The single truth source, this reservoir houses the entire application state, updating components subscribed to its changes.
- Dispatch: This is the communication channel. Components use it to send actions to the store, which then delegates them to reducers.
- Selectors: These are state extractors. By pulling out specific state fragments, they ensure components remain independent of the overarching state structure.
Implementing Redux within React:
For those eager to infuse Redux into their React applications, here’s a stepwise blueprint:
- Installation: Acquire the ‘redux’ and ‘react-redux’ lifelines via npm or yarn.
- Actions in Motion: Lay down the foundation with action types and creators, shaping the action blueprints.
- Crafting Reducers: Design the reducer functions, outlining state transitions in reaction to actions.
- Establishing the Store: Instantiate the Redux store, unifying reducers into a single overarching entity.
- Component-Store Symbiosis: Utilize ‘react-redux’s ‘connect’ function, bridging components to the Redux store.
- Action Broadcast: Empower components to dispatch actions using the store’s ‘dispatch’ conduit.
A Glimpse into Redux’s Real-world Integration:
Visualize a basic counter application as a testament to Redux’s integration within React:
// actions.js
...
// reducers.js
...
// store.js
...
// CounterComponent.js
...
In this paradigm, the Redux store supervises the counter’s state. The CounterComponent, intertwined with the store via the ‘connect’ function, transmits actions to reshape the state, with the reducer meticulously updating it.
Harnessing Redux for state management in React unlocks an array of benefits, centralizing data, and ensuring a fluid, bug-free user experience.
Unlocking Efficient React State Management with Redux
When navigating the vast sea of state management in React, Redux emerges as an anchor. Let’s delve into the manifold advantages of wielding Redux in React applications:
Top Benefits of Embracing Redux:
- Predictable & Streamlined State Transitions: Redux’s strict methodology towards state alterations guarantees easy traceability and bug resolution.
- Unified State Repository: By hosting the entire app’s state under one roof, Redux eradicates data inconsistency issues and simplifies inter-component data sharing.
- Debugging with Time Travel: Ever wished for a rewind button? Redux’s time-travel debugging feature is as close as it gets, enabling action replays to monitor state evolution.
- Middleware Mastery: Redux offers impeccable middleware integration, equipping developers to tweak actions and state transitions. This flexibility powers advanced features like action logging and asynchronous operations.
- Scalability Excellence: For mammoth applications with intricate state management, Redux stands robust, ensuring seamless data handling.
Tackling Prop Drilling with React’s Context API
As React applications expand, the intricacy of inter-component data sharing heightens. ‘Prop drilling’ rears its head, complicating state data sharing through multiple component tiers. React’s Context API provides an antidote, streamlining data sharing without the prop-passing hassle.
Deciphering Prop Drilling:
Imagine a tree. In prop drilling, data from the tree’s top has to traverse through various branches just to reach a specific leaf. In application terms, this is the repetitive and error-prone prop-passing from parent to child components.
Unveiling React’s Context API:
The Context API is React’s elegant solution for prop-drilling woes. This mechanism facilitates efficient data sharing across component hierarchies, without repetitive prop chains.
- Provider: Positioned at the component tree’s apex, the Provider holds and shares the data, ensuring accessibility to all tree descendants.
- Consumer: Components seeking shared data employ the Consumer, liberating them from the prop chain.
Implementing the Context API:
Craft a Context: Use React’s createContext function. This yields an object boasting both Provider and Consumer components.
Envelop with Provider: Surround the data-seeking components with the Provider, infusing the data as a prop.
Retrieve with Consumer: Within data-consuming components, employ the Consumer to access and utilize the shared data.
Practical Integration of Context API:
Consider a scenario with an application theme switcher, where the theme preference must be accessible across components. The Context API can manage this without resorting to prop drilling.
// ThemeContext.js
...
// App.js
...
// Header.js
...
In this paradigm, the ThemeProvider ensures theme accessibility to the Header
and Content
components. Using the Consumer, the Header
component extracts and employs the theme data, permitting users to effortlessly switch between light and dark modes.
By harnessing the power of Redux and the Context API, React developers can ensure a seamless, efficient, and maintainable approach to state management.
Enhancing React Development with the Context API and Render Props Pattern
The vast world of React development offers myriad tools and techniques to streamline application design. Among them, the Context API and Render Props pattern stand out for their efficacy and versatility.
The Context API: A Deeper Dive into Its Benefits
Navigating the maze of data sharing in React, the Context API emerges as an invaluable tool. Let’s explore its numerous benefits:
- Effortless Prop Management: The Context API cuts down on cumbersome prop drilling, enhancing code clarity and maintenance.
- Universal Data Access: Be it themes, user authentication, or localization, shared data becomes globally available to every component under the context umbrella.
- Decluttering Components: By centralizing shared data, components remain streamlined, free from the pollution of unrelated data.
- Optimized Component Reuse: Dependency on specific props for data is eradicated, fostering seamless component reuse across the application.
Render Props Pattern: A Masterstroke for Component Reusability
Component reusability in React is more than a mere advantage—it’s a development mantra. The Render Props pattern embodies this philosophy, championing component flexibility and composition.
Unraveling the Render Props Pattern:
In essence, the Render Props pattern is about passing a function (the render prop) to a component via props. This allows for the sharing of logic, state, or UI structure. The magic happens when the component invokes the render prop function instead of rendering directly, bestowing dynamic behaviour without prop drilling or intricate inheritance.
Advantages of Adopting the Render Props Pattern:
- Amplified Reusability: It nurtures the creation of versatile components, adaptable to varied scenarios.
- Distinct Responsibilities: It facilitates clear roles, enabling components to delegate responsibilities, streamlining code in the process.
- Code Sharing Without Repetition: Share intricate logic or UI structures among components without duplicating effort.
- Adaptable Rendering Behavior: Given the customization potential, components can be tailored to exact needs.
Implementing the Render Props Paradigm:
- Component Creation: Forge a component encapsulating the desired functionality.
- Render Prop Initiation: Introduce a render prop function within the component.
- Render Prop Invocation: Within the rendering phase, invoke this function, ferrying necessary data.
- Component Usage: On deployment, pass a function as the render prop. This conduit will channel shared functionality and data.
A Practical Illustration:
Envision a ‘Mouse’ component, designed to trace the cursor’s coordinates. This component, employing the Render Props pattern, shares this position data with other components:
// Mouse.js
...
// App.js
...
In this scenario, the Mouse
component captures mouse movement. The Render Props pattern comes alive when the component calls its render prop function, dispatching the mouse coordinates. The main application (App component) then employs this data, rendering it dynamically.
Render Props in the Wild:
The adaptability of the Render Props pattern finds resonance in numerous real-world applications:
- Efficient data fetching, moulded to render diverse data types.
- Crafting universal components for UI elements, including modals and tooltips.
- Sculpting higher-order components (HOCs) equipped with expansive customization avenues.
Harnessing the Context API and Render Props pattern in React projects ensures a future-ready, scalable, and efficient application design.
Mastering React Development with Higher-Order Components, Asynchronous Patterns, and Robust Testing
The dynamic landscape of React development encompasses advanced design patterns, asynchronous methodologies, and rigorous testing techniques. Let’s delve into these core concepts and their transformative impact on React applications.
Unlocking Reusability with Higher-Order Components (HOCs)
The beauty of HOCs in React lies in their potent reusability and enhanced component behaviour. Acting as component decorators, HOCs encapsulate shared logic, offering:
- Code Consolidation: Avoid repetition, embrace the DRY principle, and standardize component logic.
- Focused Functionalities: Segregate ancillary tasks like authentication or data fetching, ensuring clarity and simplicity.
- Layered Compositions: Seamlessly integrate varied functionalities with multiple HOCs.
- Design Precision: Adhere to the single responsibility principle for streamlined, easy-to-navigate components.
Practical Implementation: Enhancing a component with loading indicators, as seen in the withLoading.js
HOC perfectly exemplifies the magic of HOCs in action.
Harnessing Asynchronous Patterns for Seamless User Experiences
Asynchronous operations, especially data fetching, are indispensable for delivering real-time and dynamic content in React applications. Here’s a look at their significance:
- Parallel Execution: Asynchronous tasks run independently, ensuring uninterrupted application performance.
- Integrated Data Fetching: React’s diverse methods, from the fetch API to libraries like Axios, streamline data retrieval processes.
- Stateful Management: Properly handle different stages, from data loading to successful fetches or error occurrences.
A Working Model: The DataFetchingExample
component captures asynchronous data fetching’s essence, orchestrating loading states and successful fetches or errors seamlessly.
Bolstering React Applications with Testable Design Patterns and Practices
Guaranteeing the robustness and efficiency of React applications requires meticulous testing. By leveraging design patterns that are inherently testable and adhering to best testing practices, developers can:
- Ensure Application Integrity: Testing acts as a foundational pillar, authenticating application functionalities.
- Optimize Component Structuring: Segment components for clearer functionalities, inject dependencies and maintain the container-presentation distinction.
- Facilitate Focused Testing: Deploy design patterns like Render Props and HOCs that are inherently more testable.
- Pursue Test-driven Development: Prioritize behavior-focused tests, accurately name tests, handle edge scenarios, isolate components from external dependencies, and strike a balance across various testing types.
Diving into Practicality: The Counter
component and its corresponding test encapsulate the essence of test-driven development in React, validating interactions and expected outcomes.
Through advanced design patterns like HOCs, efficient asynchronous operations, and robust testing paradigms, React developers can craft applications that are not only functionally rich but also maintainable and scalable.
Author: Hanzala – Software Engineer
Do you have questions or need further clarification? I’m here to help. Email me anytime at hi@hanzala.co.in