Next.js 14: Revolutionary Features and Why You Should Adopt It
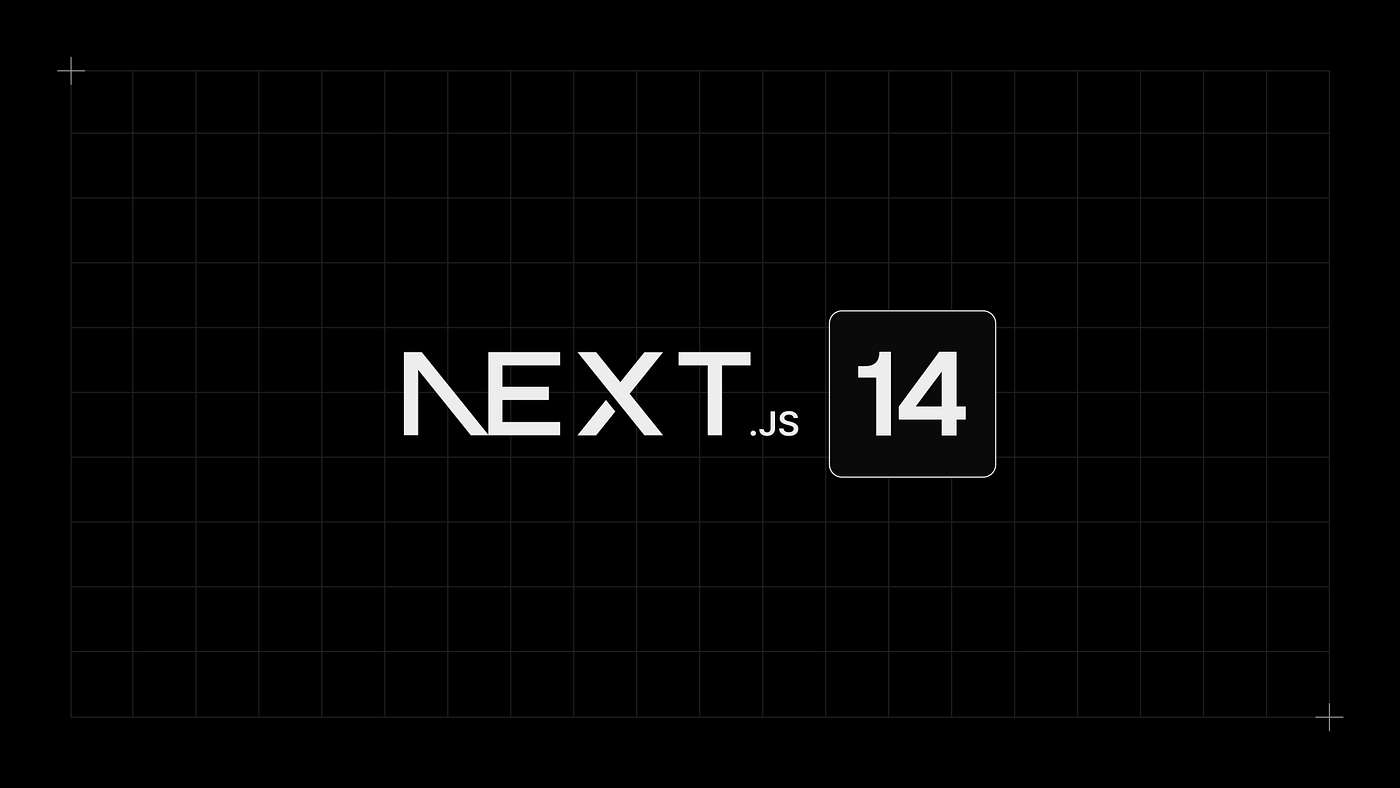
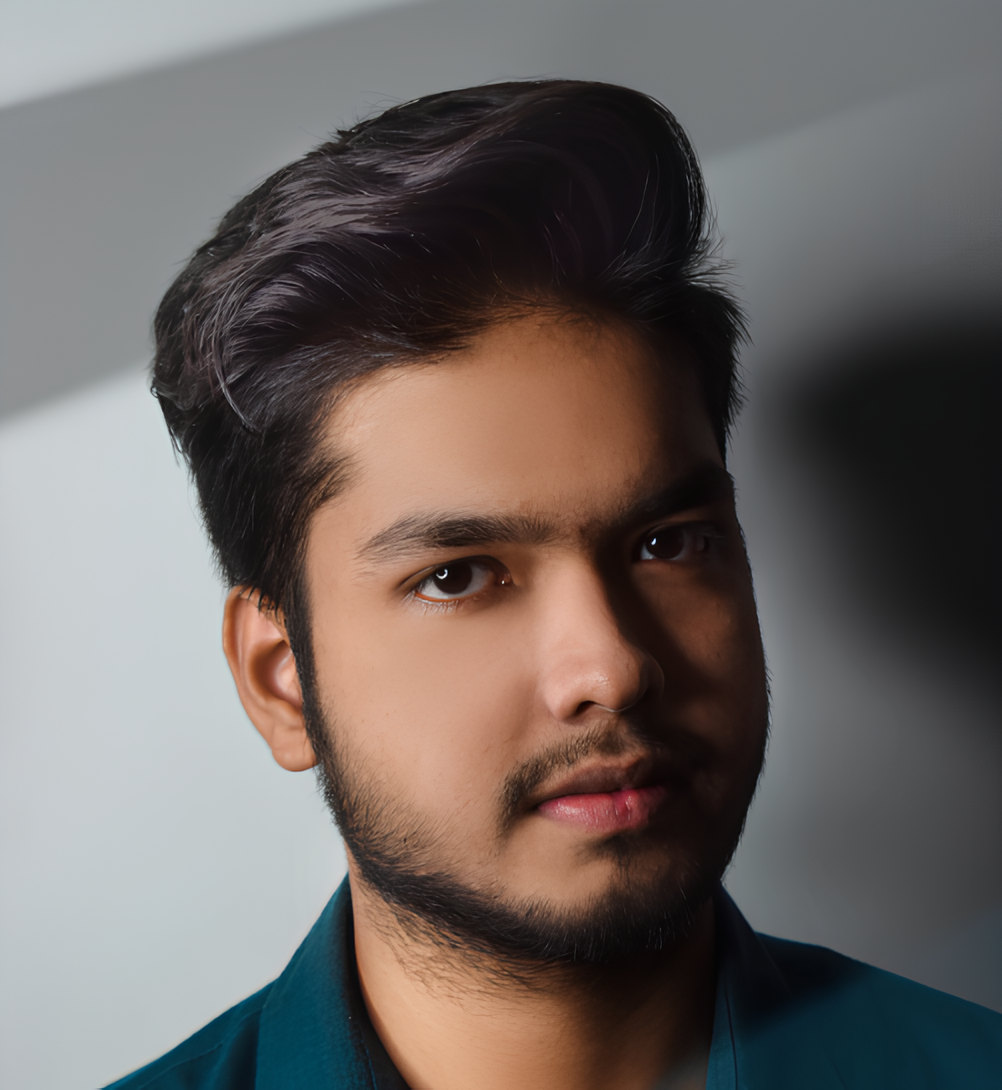
Hanzala
8 min read ⋅ Sept 13, 2024
Next.js, the popular React framework, has released its version 14, bringing a host of new features and improvements that promise to revolutionize web development. In this blog post, we’ll explore the key features of Next.js 14, discuss why you should consider using it, and how it can benefit your projects.
Key Features of Next.js 14
1. Partial Prerendering (Preview)
Next.js 14 introduces Partial Prerendering, a game-changing feature that combines static and dynamic content seamlessly.
How it works:
- Initial static shell is served instantly
- Dynamic content is streamed in on the client
- Fallback UI can be shown while loading dynamic parts
Benefits:
- Faster initial page loads
- Improved SEO with static content
- Better user experience with progressively loaded dynamic content
jsx
// Example of a partially prerendered page
export default function Page() {
return (
<div>
<h1>Welcome to My Store</h1>
<Suspense fallback={<p>Loading products...</p>}>
<Products />
</Suspense>
</div>
)
}
2. Server Actions (Stable)
Server Actions, now stable in Next.js 14, allow you to write server-side code directly in your React components.
Benefits:
- Simplifies form handling and data mutations
- Reduces client-side JavaScript
- Improves security by keeping sensitive operations server-side
jsx
// Example of a Server Action
export default function Form() {
async function handleSubmit(formData: FormData) {
'use server'
const name = formData.get('name')
await saveToDatabase(name)
}
return (
<form action={handleSubmit}>
<input type="text" name="name" />
<button type="submit">Submit</button>
</form>
)
}
3. Improved Rendering and Caching Model
Next.js 14 refines its rendering and caching model for better performance and developer experience.
Improvements:
- More intuitive caching behavior
- Enhanced static and dynamic rendering options
- Better control over revalidation and data fetching
jsx
// Example of using the new fetch options
async function getData() {
const res = await fetch('https://api.example.com/data', { next: { revalidate: 3600 } })
return res.json()
}
export default async function Page() {
const data = await getData()
return <div>{data.title}</div>
}
4. Turbopack Improvements (Beta)
Turbopack, Next.js’ Rust-based bundler, sees significant improvements in version 14.
Enhancements:
- Faster build times
- Improved compatibility with npm packages
- Better error reporting
5. Built-in SEO Support
Next.js 14 introduces new built-in components and utilities for improved SEO.
jsx
import { Metadata } from 'next'
export const metadata: Metadata = {
title: 'My Page Title',
description: 'Page description',
openGraph: {
title: 'My Open Graph Title',
description: 'Open Graph Description',
images: ['/og-image.jpg'],
},
}
export default function Page() {
return <h1>Welcome to my page</h1>
}
Why Should You Use Next.js 14?
- Performance Boost: With Partial Prerendering and improved caching, your applications will be faster than ever.
- Enhanced Developer Experience: Server Actions and improved error handling make development more intuitive and efficient.
- Better SEO Out of the Box: Built-in SEO components and static rendering capabilities improve your site’s search engine visibility.
- Simplified Full-Stack Development: Server Actions bridge the gap between frontend and backend, allowing for more integrated development.
- Future-Proof Technology: Next.js continues to evolve with web standards, ensuring your projects remain modern and efficient.
- Strong Community and Ecosystem: With its growing popularity, Next.js has a vast ecosystem of plugins, tools, and community support.
How Next.js 14 Can Help Your Projects
- Faster Time-to-Market: With improved development speed and built-in optimizations, you can launch projects faster.
- Improved User Experience: Features like Partial Prerendering ensure your users get a snappy, responsive experience.
- Scalability: Next.js 14’s architecture supports projects of all sizes, from small websites to large-scale applications.
- Cost-Effective: Improved performance and caching can lead to reduced server costs and better resource utilization.
- Better Conversion Rates: Faster load times and improved SEO can lead to better user engagement and higher conversion rates.
Getting Started with Next.js 14
To start using Next.js 14, you can create a new project or upgrade an existing one:
bash
# Create a new project
npx create-next-app@latest
# Upgrade an existing project
npm i next@latest react@latest react-dom@latest eslint-config-next@latest
Be sure to check the official Next.js documentation for detailed guides on using these new features.
Conclusion
Next.js 14 brings significant improvements that can benefit developers and businesses alike. Its focus on performance, developer experience, and SEO makes it a compelling choice for modern web development.
Whether you’re building a small personal project or a large-scale application, Next.js 14 provides the tools and optimizations to help you succeed. By adopting Next.js 14, you’re not just using a framework; you’re investing in a platform that continually evolves to meet the demands of modern web development.
Stay ahead of the curve, deliver exceptional user experiences, and build the future of the web with Next.js 14!
Hanzala — Software Developer🎓
Thank you for reading until the end. Before you go:
- Follow me X | LinkedIn | Facebook | GitHub
- Reach Out hi@hanzala.co.in