Building Advanced Data Visualizations with Highcharts and React: A Deep Dive into Custom Chart Styling
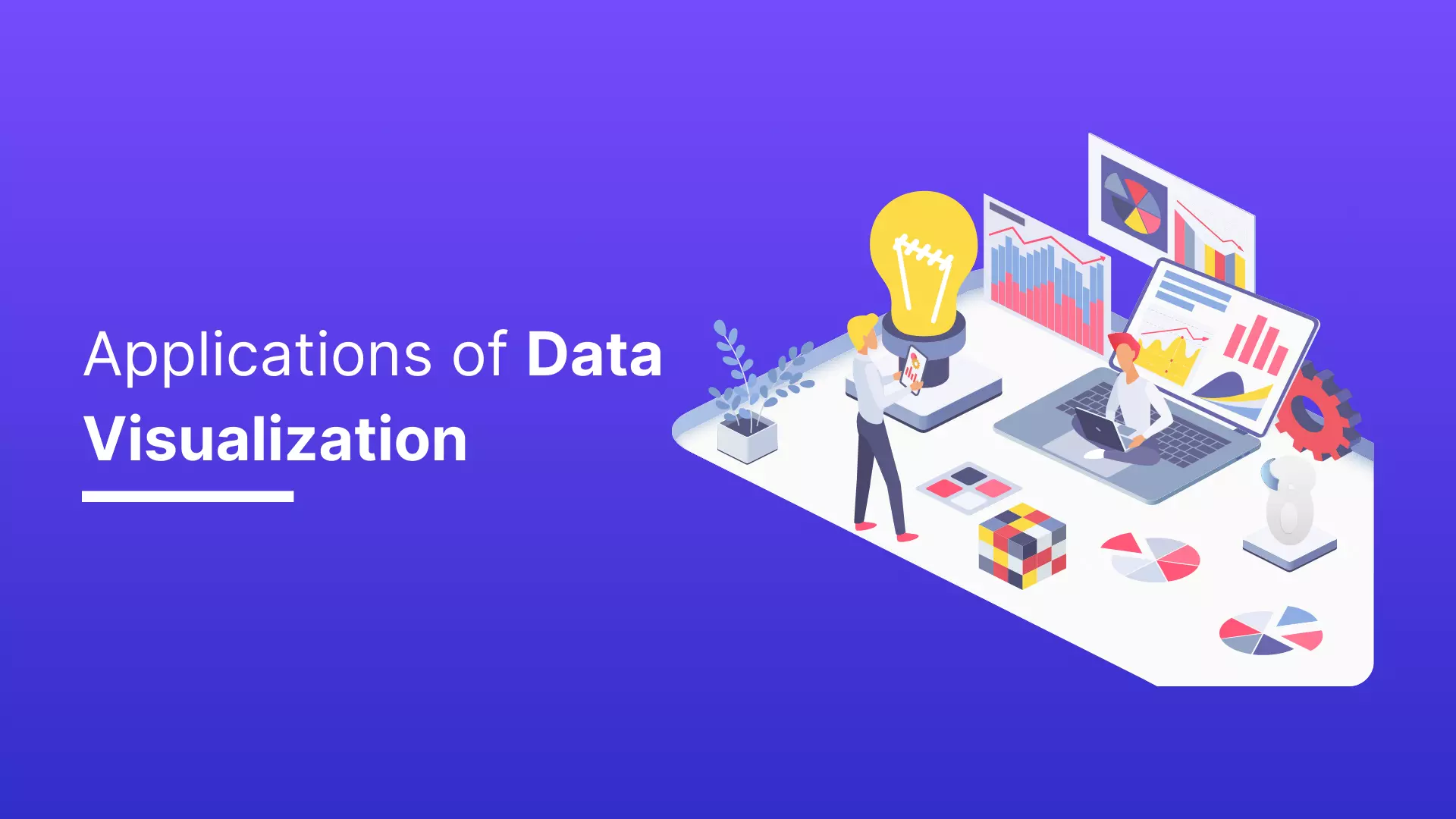
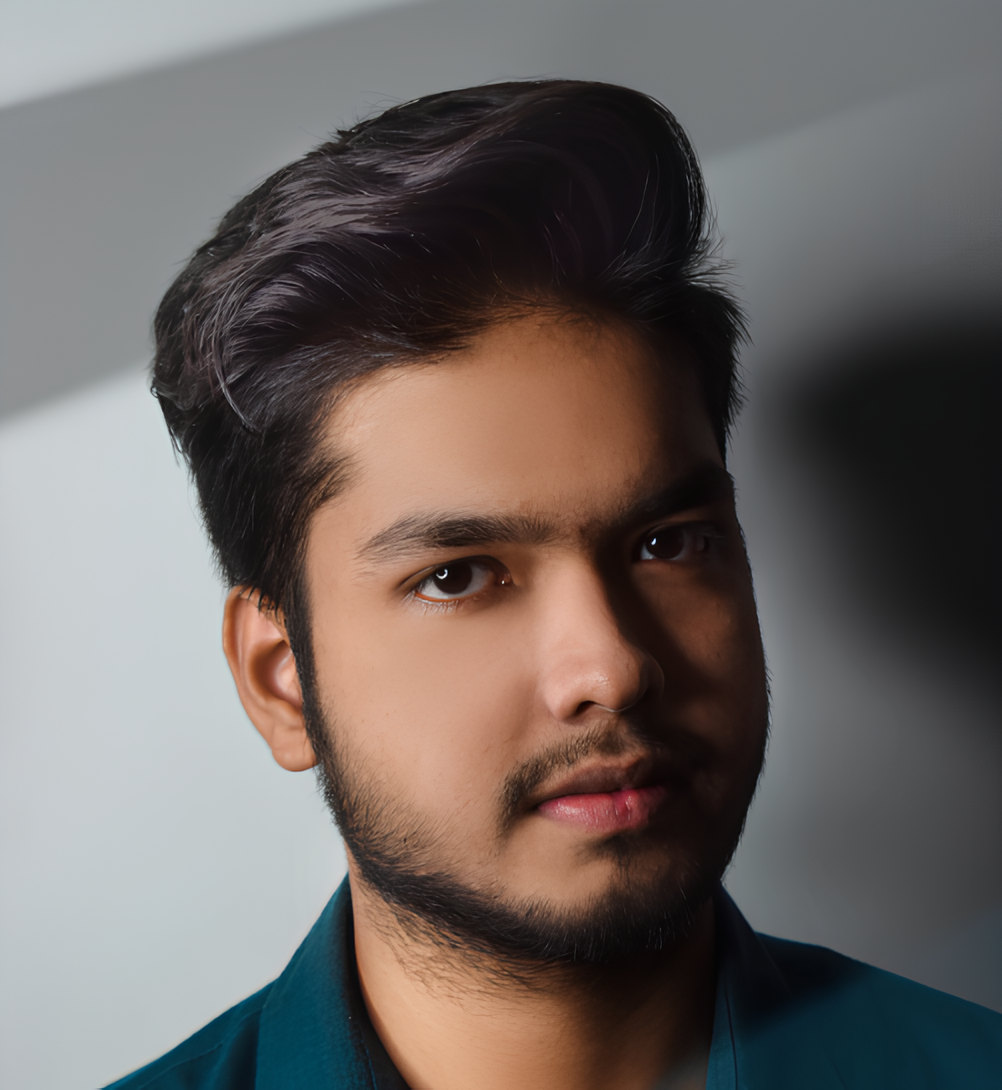
Hanzala
12 min read ⋅ Dec 6, 2024
Introduction
Data visualization is a crucial aspect of modern web applications, but creating pixel-perfect charts that meet specific design requirements can be challenging. In this comprehensive guide, we’ll explore how to build and customize complex bar charts using Highcharts with ReactJS, complete with interactive features, precise styling, and robust testing.
The Challenge
When implementing data visualizations in enterprise applications, developers often face several common challenges:
- Precise alignment of data labels with axis gridlines
- Custom styling of specific grid elements
- Interactive hover states with coordinated opacity changes
- Consistent rendering across different data sets
- Proper testing of visualization components
Let’s dive into how to solve these challenges using a production-grade approach.
Setting Up the Development Environment
First, let’s set up our project with the necessary tools:
# Create a new React project
npx create-react-app highcharts-visualization
cd highcharts-visualization
# Install dependencies
npm install highcharts highcharts-react-official @storybook/react jest @testing-library/react
Building the Base Component
Let’s create a reusable bar chart component:
import React from 'react';
import Highcharts from 'highcharts';
import HighchartsReact from 'highcharts-react-official';
const BarChart = ({ data, title }) => {
const options = {
chart: {
type: 'bar',
events: {
load() {
this.series[0].points.forEach(point => {
point.graphic.on('mouseOver', handleHover);
});
}
}
},
// ... configuration continues
};
return <HighchartsReact highcharts={Highcharts} options={options} />;
};
export default BarChart;
Custom Styling Challenges and Solutions
1. Grid Line Alignment
One of the trickiest aspects is aligning data labels perfectly with grid lines. Here’s how to achieve this:
yAxis: {
gridLineColor: '#E6E6E6',
plotLines: [{
color: '#ADADAD', // Special color for zero line
width: 1,
value: 0,
zIndex: 3
}],
labels: {
align: 'center',
x: 0
}
}
2. Interactive Hover States
Implementing sophisticated hover effects requires careful coordination:
plotOptions: {
series: {
point: {
events: {
mouseOver: function() {
const chart = this.series.chart;
chart.series[0].points.forEach(point => {
if (point !== this) {
point.graphic.attr({
opacity: 0.3
});
}
});
},
mouseOut: function() {
const chart = this.series.chart;
chart.series[0].points.forEach(point => {
point.graphic.attr({
opacity: 1
});
});
}
}
}
}
}
Testing Strategy
Unit Testing with Jest
Create comprehensive tests to ensure reliable rendering:
import { render, fireEvent, screen } from '@testing-library/react';
import BarChart from './BarChart';
describe('BarChart Component', () => {
const mockData = [
// Test data here
];
it('renders without crashing', () => {
render(<BarChart data={mockData} />);
expect(screen.getByRole('complementary')).toBeInTheDocument();
});
it('applies hover effects correctly', () => {
render(<BarChart data={mockData} />);
const firstBar = screen.getByTestId('bar-0');
fireEvent.mouseEnter(firstBar);
// Add assertions for opacity changes
});
});
Storybook Integration
Document and test visual states using Storybook:
// BarChart.stories.jsx
export default {
title: 'Components/BarChart',
component: BarChart,
argTypes: {
data: { control: 'object' },
title: { control: 'text' }
}
};
const Template = args => <BarChart {...args} />;
export const Default = Template.bind({});
Default.args = {
// Default props
};
export const WithHoverState = Template.bind({});
WithHoverState.play = async ({ canvasElement }) => {
// Simulate hover state
};
Performance Optimization
Memory Management
When working with large datasets, proper cleanup is crucial:
useEffect(() => {
return () => {
// Clean up chart instance
if (chartRef.current) {
chartRef.current.destroy();
}
};
}, []);
Render Optimization
Prevent unnecessary re-renders:
const memoizedOptions = useMemo(() => ({
// Chart options
}), [data, theme]);
Best Practices and Tips
- Accessibility: Always include ARIA labels and proper color contrast:
accessibility: {
announceNewData: true,
point: {
descriptionFormatter: function (point) {
return `${point.category}: ${point.y}%`;
}
}
}
- Responsive Design: Implement proper resize handling:
chart: {
reflow: true,
events: {
render() {
this.reflow();
}
}
}
- Error Boundaries: Implement proper error handling:
<ErrorBoundary fallback={<ChartErrorState />}>
<BarChart data={data} />
</ErrorBoundary>
Conclusion
Building professional-grade charts requires attention to detail and a thorough understanding of both the visualization library and React ecosystem. By following these patterns and best practices, you can create robust, maintainable, and visually appealing data visualizations.
Remember to:
- Always test your components thoroughly
- Consider accessibility from the start
- Optimize for performance with large datasets
- Document your components with Storybook
- Follow React best practices for state management and lifecycle handling
Additional Resources
- Highcharts API Documentation
- React Testing Library Guidelines
- Storybook Best Practices
- React Performance Optimization
By following this comprehensive approach, you’ll be well-equipped to handle complex data visualization requirements in your React applications.
Hanzala — Software Developer🎓
Thank you for reading until the end. Before you go:
- Follow me X | LinkedIn | Facebook | GitHub
- Reach Out hi@hanzala.co.in